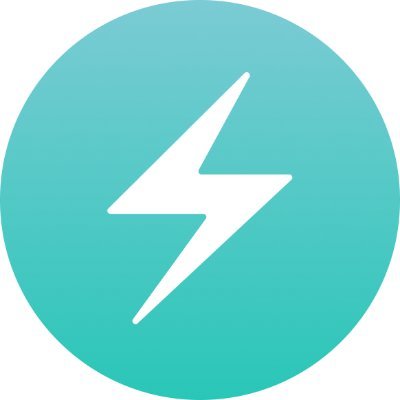
Chakra UI
The most powerful toolkit for building modern web applications.
2.5M Downloads
import { HStack, Icon, Link, Stack, Text } from "@chakra-ui/react"
import { Avatar } from "@/components/ui/avatar"
import {
HoverCardArrow,
HoverCardContent,
HoverCardRoot,
HoverCardTrigger,
} from "@/components/ui/hover-card"
import { LuChartLine } from "react-icons/lu"
const Demo = () => {
return (
<HoverCardRoot size="sm">
<HoverCardTrigger asChild>
<Link href="#">@chakra_ui</Link>
</HoverCardTrigger>
<HoverCardContent>
<HoverCardArrow />
<Stack gap="4" direction="row">
<Avatar
name="Chakra UI"
src="https://pbs.twimg.com/profile_images/1244925541448286208/rzylUjaf_400x400.jpg"
/>
<Stack gap="3">
<Stack gap="1">
<Text textStyle="sm" fontWeight="semibold">
Chakra UI
</Text>
<Text textStyle="sm" color="fg.muted">
The most powerful toolkit for building modern web applications.
</Text>
</Stack>
<HStack color="fg.subtle">
<Icon size="sm">
<LuChartLine />
</Icon>
<Text textStyle="xs">2.5M Downloads</Text>
</HStack>
</Stack>
</Stack>
</HoverCardContent>
</HoverCardRoot>
)
}
Setup
If you don't already have the snippet, run the following command to add the
hover-card
snippet
npx @chakra-ui/cli snippet add hover-card
The snippet includes component compositions based on the HoverCard
component.
Usage
import {
HoverCardArrow,
HoverCardContent,
HoverCardRoot,
HoverCardTrigger,
} from "@/components/ui/hover-card"
<HoverCardRoot>
<HoverCardTrigger />
<HoverCardContent>
<HoverCardArrow />
</HoverCardContent>
</HoverCardRoot>
Examples
Controlled
Use the open
and onOpenChange
to control the visibility of the hover card.
"use client"
import { Box, Link, Strong } from "@chakra-ui/react"
import {
HoverCardArrow,
HoverCardContent,
HoverCardRoot,
HoverCardTrigger,
} from "@/components/ui/hover-card"
import { useState } from "react"
const Demo = () => {
const [open, setOpen] = useState(false)
return (
<HoverCardRoot size="sm" open={open} onOpenChange={(e) => setOpen(e.open)}>
<HoverCardTrigger asChild>
<Link href="#">@chakra_ui</Link>
</HoverCardTrigger>
<HoverCardContent maxWidth="240px">
<HoverCardArrow />
<Box>
<Strong>Chakra</Strong> is a Sanskrit word that means disk or wheel,
referring to energy centers in the body
</Box>
</HoverCardContent>
</HoverCardRoot>
)
}
Delays
Control the open and close delays using the openDelay
and closeDelay
props.
import { Box, Link, Strong } from "@chakra-ui/react"
import {
HoverCardArrow,
HoverCardContent,
HoverCardRoot,
HoverCardTrigger,
} from "@/components/ui/hover-card"
const Demo = () => {
return (
<HoverCardRoot size="sm" openDelay={1000} closeDelay={100}>
<HoverCardTrigger asChild>
<Link href="#">@chakra_ui</Link>
</HoverCardTrigger>
<HoverCardContent maxWidth="240px">
<HoverCardArrow />
<Box>
<Strong>Chakra</Strong> is a Sanskrit word that means disk or wheel,
referring to energy centers in the body
</Box>
</HoverCardContent>
</HoverCardRoot>
)
}
Placement
Use the positioning.placement
prop to configure the underlying floating-ui
positioning logic.
import { Box, Link, Strong } from "@chakra-ui/react"
import {
HoverCardArrow,
HoverCardContent,
HoverCardRoot,
HoverCardTrigger,
} from "@/components/ui/hover-card"
const Demo = () => {
return (
<HoverCardRoot size="sm" positioning={{ placement: "top" }}>
<HoverCardTrigger asChild>
<Link href="#">@chakra_ui</Link>
</HoverCardTrigger>
<HoverCardContent maxWidth="240px">
<HoverCardArrow />
<Box>
<Strong>Chakra</Strong> is a Sanskrit word that means disk or wheel,
referring to energy centers in the body
</Box>
</HoverCardContent>
</HoverCardRoot>
)
}
Within Dialog
Here's an example of how to use the HoverCard
within a Dialog
component.
Due to the focus trap within the dialog, it's important to change the portal target from the document's body to the dialog's content.
"use client"
import { Button, Link, Stack, Text } from "@chakra-ui/react"
import {
DialogBackdrop,
DialogBody,
DialogCloseTrigger,
DialogContent,
DialogFooter,
DialogHeader,
DialogRoot,
DialogTitle,
DialogTrigger,
} from "@/components/ui/dialog"
import {
HoverCardArrow,
HoverCardContent,
HoverCardRoot,
HoverCardTrigger,
} from "@/components/ui/hover-card"
import { useRef } from "react"
const Demo = () => {
const contentRef = useRef<HTMLDivElement>(null)
return (
<DialogRoot>
<DialogBackdrop />
<DialogTrigger asChild>
<Button variant="outline">Open Dialog</Button>
</DialogTrigger>
<DialogContent ref={contentRef}>
<DialogCloseTrigger />
<DialogHeader>
<DialogTitle>Select in Dialog</DialogTitle>
</DialogHeader>
<DialogBody>
<HoverCardRoot size="sm">
<HoverCardTrigger asChild>
<Link href="#">@chakra_ui</Link>
</HoverCardTrigger>
<HoverCardContent>
<HoverCardArrow />
<Stack gap="4" direction="row">
<Stack gap="3">
<Stack gap="1">
<Text textStyle="sm" fontWeight="semibold">
Chakra UI
</Text>
<Text textStyle="sm" color="fg.muted">
The most powerful toolkit for building modern web
applications.
</Text>
</Stack>
</Stack>
</Stack>
</HoverCardContent>
</HoverCardRoot>
</DialogBody>
<DialogFooter />
</DialogContent>
</DialogRoot>
)
}
Accessibility
HoverCard should be used solely for supplementary information that is not essential for understanding the context.
It is inaccessible to screen readers and cannot be activated via keyboard, so avoid placing crucial content within it.
Props
Prop | Default | Type |
---|---|---|
closeDelay | '300' | number The duration from when the mouse leaves the trigger or content until the hover card closes. |
lazyMount | false | boolean Whether to enable lazy mounting |
openDelay | '700' | number The duration from when the mouse enters the trigger until the hover card opens. |
unmountOnExit | false | boolean Whether to unmount on exit. |
colorPalette | 'gray' | 'gray' | 'red' | 'orange' | 'yellow' | 'green' | 'teal' | 'blue' | 'cyan' | 'purple' | 'pink' | 'accent' The color palette of the component |
size | 'md' | 'xs' | 'sm' | 'md' | 'lg' The size of the component |
defaultOpen | boolean The initial open state of the hover card when it is first rendered. Use when you do not need to control its open state. | |
id | string The unique identifier of the machine. | |
ids | Partial<{
trigger: string
content: string
positioner: string
arrow: string
}> The ids of the elements in the popover. Useful for composition. | |
immediate | boolean Whether to synchronize the present change immediately or defer it to the next frame | |
onExitComplete | () => void Function called when the animation ends in the closed state | |
onOpenChange | (details: OpenChangeDetails) => void Function called when the hover card opens or closes. | |
open | boolean Whether the hover card is open | |
positioning | PositioningOptions The user provided options used to position the popover content | |
present | boolean Whether the node is present (controlled by the user) | |
as | React.ElementType The underlying element to render. | |
asChild | boolean Use the provided child element as the default rendered element, combining their props and behavior. For more details, read our Composition guide. | |
unstyled | boolean Whether to remove the component's style. |